PennController for IBEX › Forums › Support › DragDrop features › Reply To: DragDrop features
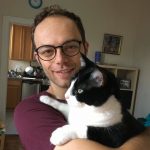
Hi,
I just realized I never addressed the previous post, so I will do it now. Unfortunately there is no built-in way to log coordinates in a DragDrop element, but it can be achieved using some javascript. The set
command of Var elements accepts javascript functions, so we can create one Var element for each pair of coordinates we want to log:
newTimer(5000).start() .wait() , newVar("manXY").set(v=>{ const bcr = getImage("man")._element.jQueryElement[0].getBoundingClientRect(); return bcr.x+';'+bcr.y; }).log(), newVar("boyXY").set(v=>{ const bcr = getImage("boy")._element.jQueryElement[0].getBoundingClientRect(); return bcr.x+';'+bcr.y; }).log(), newVar("figlistXY").set(v=>{ const bcr = getCanvas("figlist")._element.jQueryElement[0].getBoundingClientRect(); return bcr.x+';'+bcr.y; }).log() )
Now, regarding the linking of two pictures, it should be possible in theory, but I found out there are a few bugs with such chaining in the DragDrop element. We will need a few tricks to work around those bugs, namely we will remove the image of the bear once it’s placed on the flower, and replace it with a copy of it; we will need to retrieve the coordinates of the original image to do that though, which will involve some javascript
We will also need to have the bear and the flower placed on a Canvas element and make them “transparent” so that the Canvas element can be dragged onto the box:
ERRATUM: see this post on how to use a single DragDrop element instead
newText("instructions", "Put the bear on the flower").print() , newImage("bear","bear.png").size(100,100).print(), newImage("bearCopy","bear.png").size(100,100).cssContainer("pointer-events","none").css("pointer-events","none") , newCanvas("flowerCV", 200,200).add(0,0,newImage("flower","flower.png").size(200,200)).print() , newCanvas("boxCV", 300,300).add(0,0,newImage("box","box.png").size(300,300)).print() , newDragDrop("bearToFlower") .addDrag(getImage("bear")) .addDrop(getCanvas("flowerCV")) .wait() , newFunction(()=>new Promise(async r=>{ const bearBCR = getImage("bear")._element.jQueryElement[0].getBoundingClientRect(); const flowerBCR = getCanvas("flowerCV")._element.jQueryElement[0].getBoundingClientRect(); await getCanvas("flowerCV").add(bearBCR.x-flowerBCR.x,bearBCR.y-flowerBCR.y,getImage("bearCopy"))._runPromises(); r(); })).call() , getImage("bear").hidden().remove(), getImage("flower").cssContainer("pointer-events","none") , getText("instructions").text("Now put them in the box") , newDragDrop("flowerToBox") .addDrag(getCanvas("flowerCV")) .addDrop(getCanvas("boxCV")) .wait()
Let me know if you have questions
Jeremy
-
This reply was modified 1 year, 9 months ago by
Jeremy. Reason: reference to correction below