PennController for IBEX › Forums › Support › Validation of participant's ID (password) › Reply To: Validation of participant's ID (password)
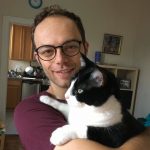
Hi,
First let me remind you that javascript is executed on the client’s side, so all the code is visible by the participant simply via clicking “View source” on the page. I would recommend using a one-way encryption method to list hashes:
const password_hashes = [ 'b94d27b9934d3e08a52e52d7da7dabfac484efe37a5380ee9088f7ace2efcde9', // hello world '4d371276d3db24d63534d530c302487492adcc1bf221387edb6836dbbf341524' // bye earth ] const digest = async message => Array.prototype.map .call( new Uint8Array( await crypto.subtle.digest("SHA-256", new TextEncoder().encode(message)) ), (x) => ("0" + x.toString(16)).slice(-2) ) .join(""); newTrial( "instruction", newText("<p>Welcome! Please fill your password into the box below.</p>").print() , newTextInput("inputID", "").center().css("margin", "1em").print() , newButton("Start my trials") .center() .print() .wait() , newFunction( "set", async function() { this.hash = await digest(document.querySelector(".PennController-inputID").value); }).call() , clear() , newFunction( "test", function () { return password_hashes.find(v=>v==this.hash); }) .testNot.is( undefined ) .failure( newText("ID not listed").print() , newButton("dummy").wait() ) , newVar("ID").global().set( getTextInput("inputID") ) )
Here I have pre-generated two hashes: one for hello world
and one for bye earth
. I have added comments but of course keeping them when actually running the experiment would defeat the whole purpose of hashing the input
You can generate the hashes to list yourself by running your experiment and, once you’re on the tab of your running experiment, open your browser’s web console and type:
digest("type the id here").then(console.log)
Replace type the id here
with an ID, and then you’ll get the hash output in the console, which you can then report in the password_hashes
array
Jeremy