PennController for IBEX › Forums › Support › Logging Results for Multiple Attempts › Reply To: Logging Results for Multiple Attempts
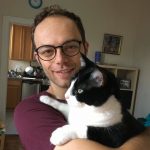
The problem is coming from the newButton commands being rerun the second time, which effectively erases the history of clicks from the element. I’ll have to work on that for future releases, but in the meantime here is a revised version of your script that should work:
const launch = (num, audio, flapChooseT, notFlapChooseT, status) => [ newAudio("flapAud" + num, audio) .play() .wait() , newText("prompt" + num, "This was...<br><br>") .settings.center() .print() , getButton("carryOn") .test.clicked() .success( getButton("flap"+num).enable().print(), getText("spacer"+num).print(), getButton("notFlap"+num).enable().print() ) .failure( newButton("flap" + num, "a flap") .print() .center() .log() .callback( getText("response" + num).text(flapChooseT), getVar("FlapScore").set(v => v + Number(status=="a flap")), getButton("flap" + num).disable(), getButton("notFlap" + num).disable(), getButton("moveOn" + num).visible() ) , newText("spacer" + num, "<br>").print() , newButton("notFlap" + num, "not a flap") .print() .center() .log() .callback( getText("response" + num).text(notFlapChooseT), getVar("FlapScore").set(v => v + Number(status == "not a flap")), getButton("flap" + num).disable(), getButton("notFlap" + num).disable(), getButton("moveOn" + num).visible() ) ) , newText("response" + num, "<p></p>") .print() , newButton("moveOn" + num, "Next") .hidden() .print() .wait() , getText("prompt" + num).remove(), getText("spacer" + num).remove(), getButton("flap" + num).remove(), getButton("notFlap" + num).remove(), getText("response" + num).remove(), getButton("moveOn" + num).remove(), ] newTrial("flapTest", newButton("carryOn").log() , newButton("launch").callback( ...launch("1", "adequateT.mp3", "<p>Correct!</p>", "<p>Incorrect.</p>", "a flap"), ...launch("7", "producingT.mp3", "<p>Incorrect.</p>", "<p>Correct!</p>", "not a flap"), ...launch("2", "bitterT.mp3", "<p>Correct!</p>", "<p>Incorrect.</p>", "a flap"), ...launch("3", "daddyT.mp3", "<p>Correct!</p>", "<p>Incorrect.</p>", "a flap"), ...launch("8", "traditionT.mp3", "<p>Incorrect.</p>", "<p>Correct!</p>", "not a flap"), ...launch("4", "mottoT.mp3", "<p>Correct!</p>", "<p>Incorrect.</p>", "a flap"), ...launch("5", "italicsT.mp3", "<p>Incorrect.</p>", "<p>Correct!</p>", "not a flap"), ...launch("6", "planetaryT.mp3", "<p>Incorrect.</p>", "<p>Correct!</p>", "not a flap"), getButton("carryOn").click() ).click() , getButton("carryOn").wait() , newText("evaluation", "Your number of correct answers: ").after(newText("").text(getVar("FlapScore"))) .print() , getVar("FlapScore") .test.is(v=>v>=5) .success( newText("<p>Good job! When you're ready, press the button below to proceed.</p>").print(), newButton("Continue").print().wait() ) .failure( newText("nope1", "<p>Given your score, we would like you to take the quiz again. Press the button below to proceed.</p>").print(), newButton("toNext1", "Continue").print().wait(), getText("nope1").remove(), getButton("toNext1").remove(), getText("evaluation").remove(), getButton("launch").click(), getButton("carryOn").wait() ) )
You’ll notice that I changed a few other things:
- I used a function in the Var’s test, which allowed me to concisely apply a “greater than” test
- I created the carryOn button first and got rid of “finally”
- I test in launch whether carryOn was clicked before, so I don’t recreate the buttons (which would erase their click history) the second time around
- I also got rid of once on the buttons because you’ll need to have them clickable again if you repeat the training phase
One note about the results file: you’ll get only one line for buttons that were never clicked, with “Never” as their timestamp, and one or two lines for the other buttons depending on how often they were clicked. Unfortunately the results lines don’t seem to appear in chronological order (despite my attempt at coding that feature, another thing I’ll need to fix for the next release) so you’ll have to reorder them when running your analyses, using the timestamps. I logged the carryOn button to make it easier to identify first-round vs second-round clicks by comparing timestamps.
Let me know if you have questions
Jeremy