PennController for IBEX › Forums › Support › Logging Results for Multiple Attempts › Reply To: Logging Results for Multiple Attempts
August 13, 2020 at 2:18 pm
#5994
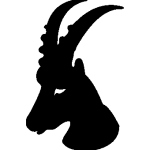
Participant
Here’s an excerpt of the code:
const launch = (num, audio, flapChooseT, notFlapChooseT, status) => [
newAudio("flapAud" + num, audio)
.play()
.wait()
,
newText("prompt" + num, "This was...<br><br>")
.settings.center()
.print()
,
newButton("flap" + num, "a flap")
.print()
.center()
.once()
.log()
.callback(getText("response" + num).text(flapChooseT), (status == "a flap" ? getVar("FlapScore").set(v => v + 1) : getVar("FlapScore").set(v => v + 0)), getButton("notFlap" + num).disable(), getButton("moveOn" + num).visible())
,
newText("spacer" + num, "<br>")
.print()
,
newButton("notFlap" + num, "not a flap")
.print()
.center()
.once()
.log()
.callback(getText("response" + num).text(notFlapChooseT), (status == "not a flap" ? getVar("FlapScore").set(v => v + 1) : getVar("FlapScore").set(v => v + 0)), getButton("flap" + num).disable(), getButton("moveOn" + num).visible())
,
newText("response" + num, "<p></p>")
.print()
,
newButton("moveOn" + num, "Next")
.hidden()
.print()
.wait()
,
getText("prompt" + num).remove(),
getText("spacer" + num).remove(),
getButton("flap" + num).remove(),
getButton("notFlap" + num).remove(),
getText("response" + num).remove(),
getButton("moveOn" + num).remove(),
]
newTrial("flapTest",
newButton("launch").callback(
...launch("1", "adequateT.mp3", "<p>Correct!</p>", "<p>Incorrect.</p>", "a flap"),
...launch("7", "producingT.mp3", "<p>Incorrect.</p>", "<p>Correct!</p>", "not a flap"),
...launch("2", "bitterT.mp3", "<p>Correct!</p>", "<p>Incorrect.</p>", "a flap"),
...launch("3", "daddyT.mp3", "<p>Correct!</p>", "<p>Incorrect.</p>", "a flap"),
...launch("8", "traditionT.mp3", "<p>Incorrect.</p>", "<p>Correct!</p>", "not a flap"),
...launch("4", "mottoT.mp3", "<p>Correct!</p>", "<p>Incorrect.</p>", "a flap"),
...launch("5", "italicsT.mp3", "<p>Incorrect.</p>", "<p>Correct!</p>", "not a flap"),
...launch("6", "planetaryT.mp3", "<p>Incorrect.</p>", "<p>Correct!</p>", "not a flap"),
getButton("carryOn").click(),
getButton("finally").click()
).click()
,
newButton("carryOn", "Next")
.print()
.hidden()
.wait()
,
newText("evaluation", "Your number of correct answers: ").after(newText("").text(getVar("FlapScore")))
.print()
,
getVar("FlapScore")
.test.is(0)
.success(newText("nope1", "<p>Given your score, we would like you to take the quiz again. Press the button below to proceed.</p>").print(), newButton("toNext1", "Continue").print().wait(), getText("nope1").remove(), getButton("toNext1").remove(), getText("evaluation").remove(), getButton("launch").click())
.failure(
getVar("FlapScore").test.is(1)
.success(newText("nope2", "<p>Given your score, we would like you to take the quiz again. Press the button below to proceed.</p>").print(), newButton("toNext2", "Continue").print().wait(), getText("nope2").remove(), getButton("toNext2").remove(), getText("evaluation").remove(), getButton("launch").click())
.failure(
getVar("FlapScore").test.is(2)
.success(newText("nope3", "<p>Given your score, we would like you to take the quiz again. Press the button below to proceed.</p>").print(), newButton("toNext3", "Continue").print().wait(), getText("nope3").remove(), getButton("toNext3").remove(), getText("evaluation").remove(), getButton("launch").click())
.failure(
getVar("FlapScore").test.is(3)
.success(newText("nope4", "<p>Given your score, we would like you to take the quiz again. Press the button below to proceed.</p>").print(), newButton("toNext4", "Continue").print().wait(), getText("nope4").remove(), getButton("toNext4").remove(), getText("evaluation").remove(), getButton("launch").click())
.failure(
getVar("FlapScore").test.is(4)
.success(newText("nope5", "<p>Given your score, we would like you to take the quiz again. Press the button below to proceed.</p>").print(), newButton("toNext5", "Continue").print().wait(), getText("nope5").remove(), getButton("toNext5").remove(), getText("evaluation").remove(), getButton("launch").click())
.failure(newText("<p>Good job! When you're ready, press the button below to proceed.</p>").print(), newButton("Continue").print().wait(), getButton("finally").click())
)
)
)
)
,
newButton("finally")
.wait()
)
We’d like to know how to log the results of the launch processes when getButton(“launch”).click() is executed near the end of the code snippet (like on line 67), i.e., how to log the button clicks in lines 10-26 the first time the launch button (line 44) is “clicked” as well as the second time. Right now it’s only logging the first round.