PennController for IBEX › Forums › Support › including attention checks and break › Reply To: including attention checks and break
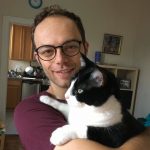
So I came up with a new function that I think is better suited to your needs: it simply picks a number of trials from a set, so in your case you can pick 40 trials from the randomized set of all the trials labeled ListeW (randomize("ListeW")), and then pick 40 other trials from that same set. The trick here is that you’ll store the set in a variable, so you can reference it when you call pick again, instead of recreating a brand new set with randomize("ListeW").
Define the pick/Pick functions the way you defined subsequence:
function Pick(set,n) { assert(set instanceof Object, "First argument of pick cannot be a plain string" ); n = Number(n); if (isNaN(n) || n<0) n = 0; this.args = [set]; set.remainingSet = null; this.run = function(arrays){ if (set.remainingSet===null) set.remainingSet = arrays[0]; const newArray = []; for (let i = 0; i < n && set.remainingSet.length; i++) newArray.push( set.remainingSet.shift() ); return newArray; } } function pick(set, n) { return new Pick(set,n); }
Then you can use it like this:
Sequence( "intro", pick(liste=randomize("ListeW"),40),"attentionCheck", pick(liste,40),"break", pick(liste,40),"attentionCheck", pick(liste,40), "end" )
As you can see I generate a randomized set of trials in the first pick function, and I store it in a javascript variable named liste, so I can reference it in the next three pick's. You could actually even create the set above the Sequence command and only reference it using the variable in all the pick functions. You just want to always use a unique javascript variable name (liste is not ideal because the generic English word list is likely to be used elsewhere, but hopefully the final e will help avoid any conflicts).
Let me know if you have questions
Jeremy