PennController for IBEX › Forums › Support › Choosing subet of items to present › Reply To: Choosing subet of items to present
July 23, 2020 at 6:22 pm
#5853
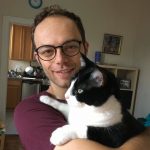
Keymaster
Hi Sam,
There probably are more optimal implementations, but how about this:
const NBLOCKS = 24; const NITEMSPERBLOCK = 12; const blocks = {}; const addItem = type => { if (!blocks.hasOwnProperty(type)) blocks[type] = [...new Array(NBLOCKS)].map((v,i)=>Object({id:i, n:0})); let freeblocks = blocks[type].filter(v=>v.n<NITEMSPERBLOCK); if (!freeblocks.length) return console.error("# of items in table does not match config"); let r = Math.floor(Math.random()*freeblocks.length); freeblocks[r].n++; return type+"-"+freeblocks[r].id; } Sequence( rshuffle("Test-0","Train-0"),"pause", rshuffle("Test-1","Train-1"),"pause", rshuffle("Test-2","Train-2"),"pause", rshuffle("Test-3","Train-3"),"pause", rshuffle("Test-4","Train-4"),"pause", rshuffle("Test-5","Train-5"),"pause", rshuffle("Test-6","Train-6"),"pause", rshuffle("Test-7","Train-7"),"pause", rshuffle("Test-8","Train-8"),"pause", rshuffle("Test-9","Train-9"),"pause", rshuffle("Test-10","Train-10"),"pause", rshuffle("Test-11","Train-11"),"pause", rshuffle("Test-12","Train-12"),"pause", rshuffle("Test-13","Train-13"),"pause", rshuffle("Test-14","Train-14"),"pause", rshuffle("Test-15","Train-15"),"pause", rshuffle("Test-16","Train-16"),"pause", rshuffle("Test-17","Train-17"),"pause", rshuffle("Test-18","Train-18"),"pause", rshuffle("Test-19","Train-19"),"pause", rshuffle("Test-20","Train-20"),"pause", rshuffle("Test-21","Train-21"),"pause", rshuffle("Test-22","Train-22"),"pause", rshuffle("Test-23","Train-23") ) newTrial("pause", newButton("Take a break").print().wait() ) Template( "myTable" , row => newTrial( addItem(row.Type) , newButton(row.Word).print().wait() ) )
This will randomly assign each of the items from your table to one of 24 (NBLOCKS) type-specific blocks of 12 (NITEMSPERBLOCK) items each. You can then write a Sequence where you randomly shuffle the items in those randomly-generated blocks, and separate each block by a pause trial.
Jeremy