PennController for IBEX › Forums › Support › Repeat Trial After Feedback › Reply To: Repeat Trial After Feedback
April 13, 2020 at 3:32 pm
#5039
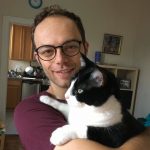
Keymaster
Hi Farzaneh,
Yeah, PCIbex is not great at handling loops at the moment, I should work on a solution for that. In the meantime, what you can do is first create all the elements you will manipulate at the beginning of your trial, and put the bit that needs to be re-executed upon failure in an array, so you can reference it multiple times, like this:
// We'll refer to this sequence of commands multiple times let launch = [ newTimer(500) .start() .wait() , getAudio("BeepBeep.mp3") .play() .wait() , newTimer(500) .start() .wait() , getAudio("PracSHSR.mp3") .play() , getImage("one") .size(380,380) .css("border", "solid 1px black") , getImage("two") .size(380,380) .css("border", "solid 1px black") , getImage("three") .size(380,380) .css("border", "solid 1px black") , getCanvas("images") .add( 0 , 0, getImage("one") ) .add( 400 , 0 , getImage("two") ) .add( 800, 0, getImage("three") ) .print() , getAudio("PracSHSR.mp3") .wait() , getSelector("choice").enable() ] newTrial( // Create your elements first newAudio("BeepBeep.mp3"), newAudio("PracSHSR.mp3"), newAudio("Beep.mp3") , newImage("one", "Man.png"), newImage("two", "Boy.png"), newImage("three", "Girl.png") , newCanvas("images", 1200 , 400) , newText("positiveFeedback", "Good job!"), newText("negativeFeedback", "Wrong answer. Try again.") , newSelector("choice") , // Now execute the sequence of commands above ...launch , getSelector("choice") .add(getImage ("one"), getImage ("two"), getImage ("three") ) .keys ("F", "J", "T") .log() // Only release 'wait' if correct choice .wait( getSelector("choice").test.selected( getImage("two") ) .failure( // If wrong choice... getText("negativeFeedback") .center() .print() , newTimer(2000).start().wait() , getText("negativeFeedback").remove() , // Make sure to disable selection again getSelector("choice").disable() , // And take the Canvas off the page getCanvas("images").remove() , // Now re-execute the sequence of commands above ...launch ) ) .disable() , getText("positiveFeedback") .center() .print() , newTimer(2000) .start() .wait() , getAudio("Beep.mp3") .play() .wait("first") , newTimer(1000) .start() .wait() )
Let me know if you have questions