PennController for IBEX › Forums › Support › Question about assigning conditions, groups + randomization › Reply To: Question about assigning conditions, groups + randomization
June 23, 2023 at 7:20 am
#10702
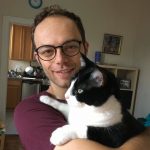
Keymaster
Hi,
There are many ways to go about this, but here is a suggestion. You can first use Template
to read the CSV table into a javascript dictionary, then manipulate that dictionary in a later Template
command to create three items per row, but only in the NEG or POS condition for each pair. Here’s on way of doing it:
// Note: Template gives us access to the content of the CSV file, but it executes the function it is passed // only after this whole script file has been executed, this is why we need to place our code inside Template const targets = {} // store the rows from the CSV file into a javascript dictionary Template("target_test.csv", row => { // the dictionary lists all the same-pair items under the same key if (targets[row.pair]===undefined) targets[row.pair] = {POS:[],NEG:[]}; targets[row.pair][row.target_polarity].push(row); return {}; // we're not actually generating trials here: return an empty object }) AddTable("dummy", "a,b\rc,d") // Dummy one-row table to execute some code from Template once after the code above Template("dummy", () => { const targetKeys = Object.keys(targets); fisherYates(targetKeys); // shuffle the references to the pairs let new_targets = []; // this will contain half the items (only POS or only NEG for each pair) for (let i=0; i<targetKeys.length; i++) // keep the POS rows for the first half, the NEG rows for the second half new_targets.push( ...targets[targetKeys[i]][ i<targetKeys.length/2 ? "POS" : "NEG"] ); // Create three items per row that we kept new_targets = new_targets.map(t=> [ [t['construction 1'],t['c1 question']],[t['construction 2'],t['c2 question']],[t['construction 3'],t['c3 question']] ].map(t2=> ["experiment_"+t.pair,"PennController",newTrial( newText([t.context_adj,t.target_adj,t.target_polarity,t2[0],t2[1]].join("<br>")).print() , newButton("Next").print().wait() )] ) // this map returns an array of 3 trials ).flat(); // flatten the array to have all the trials at the root, instead of having a series of arrays of 3 trials // Shuffle new_targets as long as we can find three items in a row that come from the same pair while (new_targets.find( (v,i)=>i<(new_targets.length-2) && v[0].split('_')[1]==new_targets[i+1][0].split('_')[1] && v[0].split('_')[1]==new_targets[i+2][0].split('_')[1] )) fisherYates(new_targets); window.items = new_targets; // now add the trials to the experiment's items return {}; // we added the items manually above: return an empty object from Template })
Jeremy